Coords 1: Getting Started with astropy.coordinates¶
Authors¶
Erik Tollerud, Kelle Cruz, Stephen Pardy, Stephanie T. Douglas
Learning Goals¶
Create
astropy.coordinates.SkyCoord
objects using names and coordinatesUse SkyCoord objects to become familiar with object oriented programming (OOP)
Interact with a
SkyCoord
object and access its attributesUse a
SkyCoord
object to query a database
Keywords¶
coordinates, OOP, file input/output
Summary¶
In this tutorial, we’re going to investigate the area of the sky around the picturesque group of galaxies named “Hickson Compact Group 7,” download an image, and do something with its coordinates.
Imports¶
[1]:
# Python standard-library
from urllib.parse import urlencode
from urllib.request import urlretrieve
# Third-party dependencies
from astropy import units as u
from astropy.coordinates import SkyCoord
from IPython.display import Image
Describing on-sky locations with coordinates
¶
The SkyCoord
class in the astropy.coordinates
package is used to represent celestial coordinates. First, we’ll make a SkyCoord object based on our object’s name, “Hickson Compact Group 7” or “HCG 7” for short. Most astronomical object names can be found by SESAME, a service which queries Simbad, NED, and VizieR and returns the object’s type and its J2000 position. This service can be used via the SkyCoord.from_name()
class
method:
[2]:
# initialize a SkyCood object named hcg7_center at the location of HCG 7
hcg7_center = SkyCoord.from_name('HCG 7')
Note that the above command requires an internet connection. If you don’t have one, execute the following line instead:
[ ]:
# uncomment and run this line if you don't have an internet connection
# hcg7_center = SkyCoord(9.81625*u.deg, 0.88806*u.deg, frame='icrs')
[3]:
type(hcg7_center)
[3]:
astropy.coordinates.sky_coordinate.SkyCoord
Show the available methods and attributes of the SkyCoord object we’ve created called hcg7_center
[4]:
dir(hcg7_center)
[4]:
['T',
'__abstractmethods__',
'__bool__',
'__class__',
'__delattr__',
'__dict__',
'__dir__',
'__doc__',
'__eq__',
'__format__',
'__ge__',
'__getattr__',
'__getattribute__',
'__getitem__',
'__gt__',
'__hash__',
'__init__',
'__init_subclass__',
'__iter__',
'__le__',
'__len__',
'__lt__',
'__module__',
'__ne__',
'__new__',
'__reduce__',
'__reduce_ex__',
'__repr__',
'__setattr__',
'__sizeof__',
'__str__',
'__subclasshook__',
'__weakref__',
'_abc_impl',
'_apply',
'_extra_frameattr_names',
'_sky_coord_frame',
'altaz',
'apply_space_motion',
'barycentricmeanecliptic',
'barycentrictrueecliptic',
'cache',
'cartesian',
'cirs',
'contained_by',
'copy',
'custombarycentricecliptic',
'data',
'dec',
'default_differential',
'default_representation',
'diagonal',
'differential_type',
'directional_offset_by',
'distance',
'equinox',
'fk4',
'fk4noeterms',
'fk5',
'flatten',
'frame',
'frame_attributes',
'frame_specific_representation_info',
'from_name',
'from_pixel',
'galactic',
'galacticlsr',
'galactocentric',
'galcen_coord',
'galcen_distance',
'galcen_v_sun',
'gcrs',
'geocentricmeanecliptic',
'geocentrictrueecliptic',
'get_constellation',
'get_frame_attr_names',
'get_representation_cls',
'get_representation_component_names',
'get_representation_component_units',
'guess_from_table',
'has_data',
'hcrs',
'heliocentriceclipticiau76',
'heliocentricmeanecliptic',
'heliocentrictrueecliptic',
'icrs',
'info',
'is_equivalent_frame',
'is_frame_attr_default',
'is_transformable_to',
'isscalar',
'itrs',
'location',
'lsr',
'match_to_catalog_3d',
'match_to_catalog_sky',
'name',
'ndim',
'obliquity',
'obsgeoloc',
'obsgeovel',
'obstime',
'obswl',
'pm_dec',
'pm_ra_cosdec',
'position_angle',
'precessedgeocentric',
'pressure',
'proper_motion',
'ra',
'radial_velocity',
'radial_velocity_correction',
'ravel',
'realize_frame',
'relative_humidity',
'replicate',
'replicate_without_data',
'represent_as',
'representation',
'representation_component_names',
'representation_component_units',
'representation_info',
'representation_type',
'reshape',
'roll',
'search_around_3d',
'search_around_sky',
'separation',
'separation_3d',
'set_representation_cls',
'shape',
'size',
'skyoffset_frame',
'spherical',
'spherical_offsets_to',
'sphericalcoslat',
'squeeze',
'supergalactic',
'swapaxes',
'take',
'temperature',
'to_pixel',
'to_string',
'transform_to',
'transpose',
'v_bary',
'velocity',
'z_sun']
Show the RA and Dec.
[6]:
print(hcg7_center.ra, hcg7_center.dec)
print(hcg7_center.ra.hour, hcg7_center.dec.hour)
9d48m58.5s 0d53m17s
0.6544166666666668 0.05920370400000001
We see that, according to SESAME, HCG 7 is located at ra = 9.849 deg and dec = 0.878 deg.
This object we’ve just created has various useful ways of accessing the information contained within it. In particular, the ra
and dec
attributes are specialized Quantity objects (actually, a subclass called Angle, which in turn is subclassed by Latitude and
Longitude). These objects store angles and provide pretty representations of those angles, as well as some useful attributes to quickly convert to common angle units:
[7]:
type(hcg7_center.ra), type(hcg7_center.dec)
[7]:
(astropy.coordinates.angles.Longitude, astropy.coordinates.angles.Latitude)
[8]:
hcg7_center.ra, hcg7_center.dec
[8]:
(<Longitude 9.81625 deg>, <Latitude 0.88805556 deg>)
[9]:
hcg7_center
[9]:
<SkyCoord (ICRS): (ra, dec) in deg
(9.81625, 0.88805556)>
[13]:
hcg7_center.ra
[13]:
SkyCoord will also accept string-formatted coordinates either as separate strings for RA/Dec or a single string. You’ll need to give units, though, if they aren’t part of the string itself.
[11]:
SkyCoord('0h39m15.9s', '0d53m17.016s', frame='icrs')
[11]:
<SkyCoord (ICRS): (ra, dec) in deg
(9.81625, 0.88806)>
[12]:
hcg7_center.ra.hour
[12]:
0.6544166666666668
Download an image¶
Now that we have a SkyCoord
object, we can try to use it to access data from the Sloan Digitial Sky Survey (SDSS). Let’s start by trying to get a picture using the SDSS image cutout service to make sure HCG 7 is in the SDSS footprint and has good image quality.
This requires an internet connection, but if it fails, don’t worry: the file is included in the repository so you can just let it use the local file'HCG7_SDSS_cutout.jpg'
, defined at the top of the cell.
[14]:
# tell the SDSS service how big of a cutout we want
im_size = 12*u.arcmin # get a 12 arcmin square
im_pixels = 1024
cutoutbaseurl = 'http://skyservice.pha.jhu.edu/DR12/ImgCutout/getjpeg.aspx'
query_string = urlencode(dict(ra=hcg7_center.ra.deg,
dec=hcg7_center.dec.deg,
width=im_pixels, height=im_pixels,
scale=im_size.to(u.arcsec).value/im_pixels))
url = cutoutbaseurl + '?' + query_string
# this downloads the image to your disk
urlretrieve(url, 'HCG7_SDSS_cutout.jpg')
[14]:
('HCG7_SDSS_cutout.jpg', <http.client.HTTPMessage at 0x1b9798bf248>)
[15]:
Image('HCG7_SDSS_cutout.jpg')
[15]:
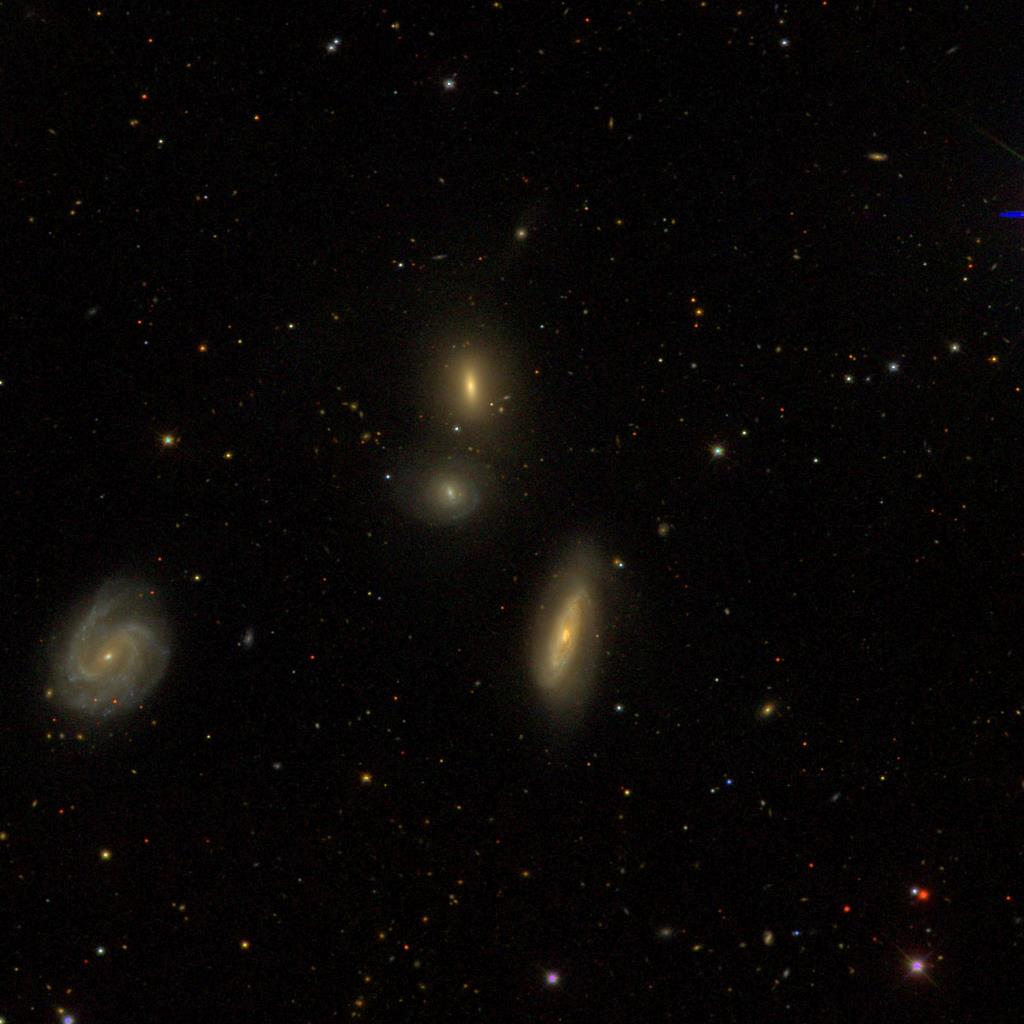
Very pretty!
The saga of HCG 7 continues in Coords 2: Transforming between coordinate systems.
Exercises¶
Exercise 1: NGC 2392, The Eskimo Nebula¶
Create a SkyCoord
of some other astronomical object you find interesting. Using only a single method/function call, get a string with the RA/Dec in the form ‘HH:MM:SS.S DD:MM:SS.S’. Check your answer against an academic paper or a website like SIMBAD that will show you sexigesimal coordinates for the object.
(Hint: SkyCoord.to_string()
might be worth reading up on.)
[16]:
Eskimo_center = SkyCoord.from_name('NGC 2392')
Eskimo_center
[16]:
<SkyCoord (ICRS): (ra, dec) in deg
(112.29486213, 20.91179959)>
[17]:
Eskimo_center.ra, Eskimo_center.dec
[17]:
(<Longitude 112.29486213 deg>, <Latitude 20.91179959 deg>)
Exercise 2¶
Now get an image of that object from the Digitized Sky Survey and download it and/or show it in the notebook. Bonus points if you figure out the (one-line) trick to get it to display in the notebook without ever downloading the file yourself.
(Hint: STScI has an easy-to-access copy of the DSS. The pattern to follow for the web URL is http://archive.stsci.edu/cgi-bin/dss_search?f=GIF&ra=RA&dec=DEC
.)
[ ]:
[25]:
# tell the SDSS service how big of a cutout we want
im_size = 8*u.arcmin # get a 12 arcmin square
im_pixels = 1024
cutoutbaseurl = 'http://skyservice.pha.jhu.edu/DR12/ImgCutout/getjpeg.aspx'
query_string = urlencode(dict(ra=Eskimo_center.ra.deg,
dec=Eskimo_center.dec.deg,
width=im_pixels, height=im_pixels,
scale=im_size.to(u.arcsec).value/im_pixels))
url = cutoutbaseurl + '?' + query_string
# this downloads the image to your disk
urlretrieve(url, 'Eskimo_SDSS_cutout.8.jpg')
[25]:
('Eskimo_SDSS_cutout.8.jpg', <http.client.HTTPMessage at 0x1b9798f09c8>)
[26]:
Image('Eskimo_SDSS_cutout.8.jpg')
[26]:
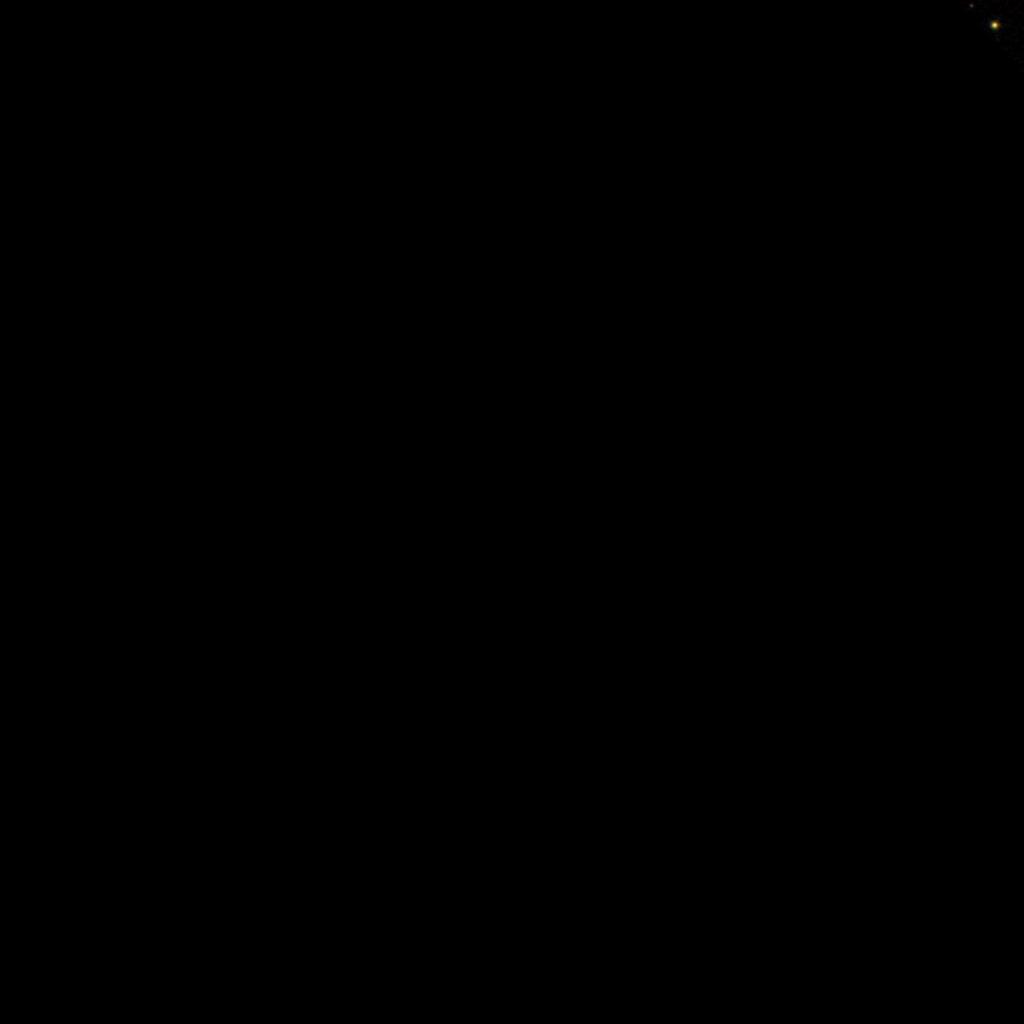
[24]:
Image('Eskimo_SDSS_cutout.38arcmin.jpg')
[24]:
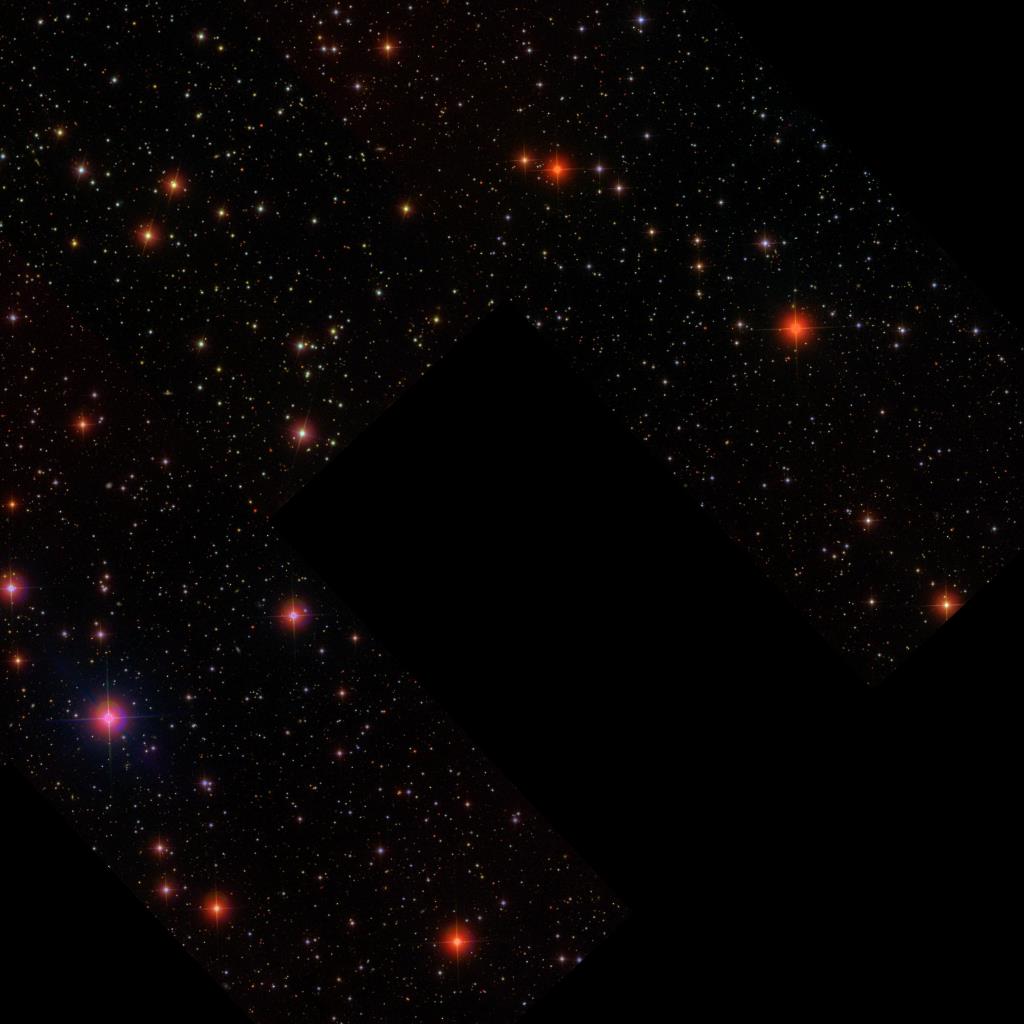
[ ]: